Simple Components
A simple Cat Component
This content:
pages/intro-to-components.mdx
import Cat from '@/components/cat'
<Cat height={200} width={200} />
leads to this image:
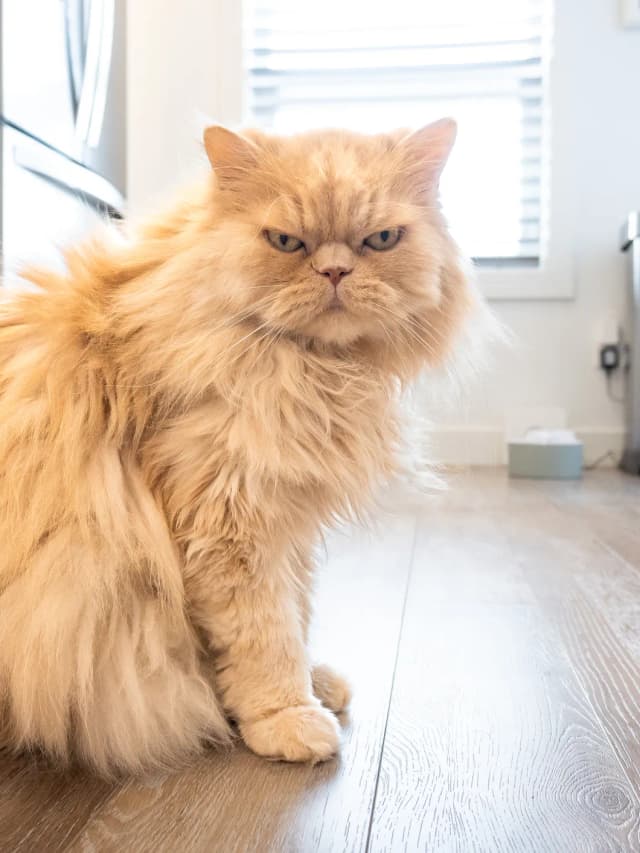
The content of the cat
component is:
components/cat.jsx
import Image from 'next/image'
function Cat({ width=500, height=500, src="/images/cat.webp" }) {
return (
<Image src={src} alt="a cat" width={width} height={height} />
)
}
export default Cat
Let us call the component again:
<Cat src="/images/cats.webp" height={400} width={400} />
This is the result:
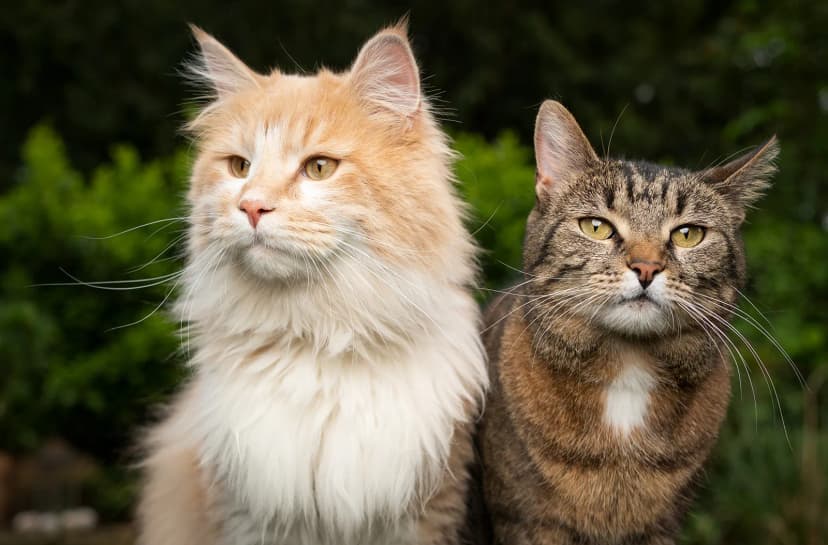
Components with State
This mdx code:
import { useState } from 'react'
{/* Import CSS modules */}
import styles from '../components/counters.module.css'
export const Counter = () => {
const [count, setCount] = useState(4);
return (
<div>
<button onClick={() => setCount(count + 1)} className={styles.counter}>Clicked {count} times</button>
</div>
);
};
<Counter/>
produces this output:
External Component
The code:
pages/intro-to-components.mdx
import Counters from '../components/counters.jsx'
looks like:
<Counters />
looks like: